This article shows how to plot data from CSV file.
Contents
◆How to plot data from CSV file
- Using matplotlib and csv module
- Using matplotlib and pandas
◆[Supplement]How to plot data from CSV file by using pandas
This is the csv file of example code.
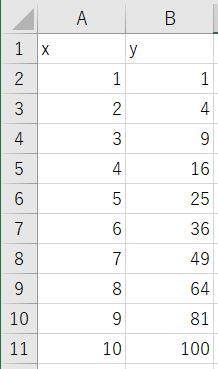
sponsored link
How to plot data from CSV file by using matplotlib and csv module
I show how to plot data from CSV file by using matplotlib and csv module.
This is the example code.
The data in the csv file is read by using the "csv module", a standard python module.
import csv
import numpy as np
import matplotlib.pyplot as plt
csv_path = r'test\test.csv'
rows = []
with open(csv_path) as f:
reader = csv.reader(f)
rows = [row for row in reader]
header = rows.pop(0)
data = np.float_(np.array(rows).T)
fig, ax = plt.subplots()
ax.plot(data[0], data[1], linestyle='solid', marker='o')
ax.set_xlabel(header[0])
ax.set_ylabel(header[1])
plt.show()
The above code generates the following graph.
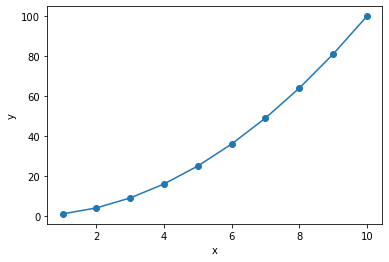
> with open(csv_path) as f:
> reader = csv.reader(f)
> rows = [row for row in reader]
The data in the csv file is read using the "csv module".
The csv.reader( ) is used to read the csv file, which returns an "reader object".
Using comprehension, data is extracted row by row from the "reader object" to create a new list.
> header = rows.pop(0)
The 0th element of the csv data is retrieved by using "pop method".
> np.float_(np.array(rows).T)
The data is transformed into a form that can be plotted in a graph.
np.array(rows).T : After transforming into numpy type, it is transposed.
np.float_( ) : Transforms to floating type.
> ax.plot(data[0], data[1], linestyle='solid', marker='o')
The data is passed to the graph.
> ax.set_xlabel(header[0])
> ax.set_ylabel(header[1])
X-axis and Y-axis labels are specified.
How to use of comprehension is explained in this article.
How to use of "pop method" of a list is explained in this article.
Remove Elements from List [del, remove, pop, clear]
sponsored link
How to plot data from CSV file by using matplotlib and pandas
Next, I show how to read csv data by using "pandas" and draw a graph by using "matplotlib".
This is the example code.
The data in the csv file is read by using "pandas.read_csv" function.
import pandas as pd
import matplotlib.pyplot as plt
path_csv = r'test\test.csv'
df_csv = pd.read_csv(path_csv)
data_x = df_csv[df_csv.columns[0]]
data_y = df_csv[df_csv.columns[1]]
fig, ax = plt.subplots()
ax.plot(data_x, data_y, linestyle='solid', marker='o')
ax.set_xlabel(df_csv.columns[0])
ax.set_ylabel(df_csv.columns[1])
plt.show()
The above code generates the following graph.
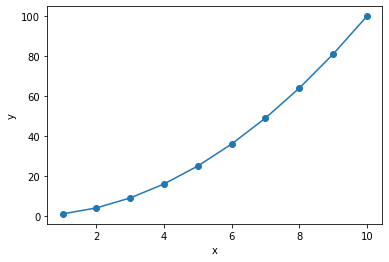
> df_csv = pd.read_csv(path_csv)
The data in the csv file is read using the "pandas.read_csv" function.
> data_x = df_csv[df_csv.columns[0]]
> data_y = df_csv[df_csv.columns[1]]
The data is transformed into a form that can be plotted in a graph.
The "df_csv.columns" function is used to get the column names of the DataFrame.
The data corresponding to each of the X and Y axes is extracted by specifying "df_csv[ 'column name' ]".
sponsored link
[Supplement]How to plot data from CSV file by using pandas
As a supplement, I show how to draw a graph from a csv file by using "pandas".
Pandas also provides a method for drawing graphs.
This method makes it easy to draw graphs.
This is the example code.
import pandas as pd
path_csv = r'test\test.csv'
df_csv = pd.read_csv(path_csv)
fig = plt.figure()
ax = fig.add_subplot(1, 1, 1)
df_csv.plot(ax=ax, x=df_csv.columns[0], y=df_csv.columns[1], marker='o')
The above code generates the following graph.

> df_csv.plot(ax=ax, x=df_csv.columns[0], y=df_csv.columns[1], marker='o')
The "df.plot( ) method" is used to draw a graph.
sponsored link